How to transfer data securely using JWT in ServiceNow
In this article I will take you through from certificate creation till generating an encrypted JWT payload in ServiceNow. We can use this JWT payload for secure data transfer.
There are many articles and blogs on what JWT is and how to use it in ServiceNow if we already have a certificate and a key. However, if you want to do both of these by yourself then this article is for you.
Abbreviations used
JKS – Java Key Store
JWT – JSON Web Token
SSO – Single Sign On
PKCS12 – A cryptographic archive file format
References
Here is the 4 steps approach to achieve this
Step1: Arrange all the prerequisites
Step2: Generate a JKS file
Step3: Configure JWT in ServiceNow
Step4: Generate a JWT for a sample payload
After the above 4 steps you will have a JWT ready, and you can transfer this data based on your requirement.
Step1: Arrange all the prerequisites
In this topic we are generating our own certificate and keys to configure JWT in ServiceNow. To achieve this, below are the 3 prerequisites.
- Multi provider SSO plugin – if not already installed in your instance, then search plugins with id com.snc.integration.sso.multi.ui and install
- Install openssl on your computer
- Openssl - A robust, commercial-grade, full-featured toolkit for general-purpose cryptography and secure communication.
- Use this reference link to install or any other source from your Google search results
- Java Keytool
- Keytool is a certificate management utility included with Java.
- Install JDK on your computer and Java Keytool will be installed automatically along with this
Step2: Generate a JKS file
We are generating JKS file using below 3 step approach
- Generate a key & a certificate
- Use the below command in Terminal(in Mac OS) or CMD(in Windows)
- openssl req -new -days 365 -nodes -x509 -keyout dummykey.key -out dummycert.crt
- In the above command dummykey & dummycert are the sample names and you can give whatever you want. Answer the questions in the Terminal, it is the standard procedure.
- Combine the generated key & certificate into a PKCS12 file
- openssl pkcs12 -export -in dummycert.crt -inkey dummykey.key -certfile dummycert.crt -out dummycert.p12
- Convert PKCS12 file to JKS
- keytool -importkeystore -srckeystore dummycert.p12 -srcstoretype pkcs12 -destkeystore dummycert.jks -deststoretype JKS
Step3: Configure JWT in ServiceNow
- Create Certificate (X.509) with below values
- Attach the above created dummycert.jks file
- Name: any name
- Type: Java Key Store and Save
- Create JWT Key with below values
- Name: any name
- SigningKey: Password@123 (or any other which you used to generate jks)
- Signing KeyStore: Select the above step value
- Create JWT Provider with below value
- Name: any name
- Signing Configuration: above created step value
Step4: Generate a JWT for a sample payload
Below is a sample script to generate JWT of a sample payload using GlideJWTAPI
Script
var jwtAPI = new sn_auth.GlideJWTAPI(); var headerJSON = { typ: "JWT", alg: "RSA256"}; var header = JSON.stringify(headerJSON); var payloadJSON = { "employeeNumber": gs.getUser().getID()}; var payload = JSON.stringify(payloadJSON); var jwtProviderSysId = "0d6ed1f02fe011105123b2e72799b638"; var jwt = jwtAPI.generateJWT(jwtProviderSysId, header, payload); gs.info("JWT:" + jwt);
Output
eyJhbGciOiJSUzI1NiIsInR5cCI6IkpXVCJ9.eyJleHAiOjE2NTY5OTIxNjEsImlhdCI6MTY1Njk5MjEwMSwianRpIjoiNzAxOGQxNDctNDU1ZC00YmY4LWFjYjctM2MxOTBmMTQ2MWU3IiwiZW1wbG95ZWVOdW1iZXIiOiI2ODE2Zjc5Y2MwYTgwMTY0MDFjNWEzM2JlMDRiZTQ0MSJ9.GIgPASK0tkcLP8GC7WCdE4NaqB_d_Rl1nunB1P2tZIDKc_6by_dTC1nrTAvjuGlntam-2iqF8LhWa6VF5zQXRw-j0gUnNVBjDTyyGrmNf4tNUtBuFt_4eAb-e3dTJEuLvXteXSWK-Rqcbvy3phfRUnzca2LZkFJQj3c890YndGlHlQRoJGk7tQYiMYF8dkrOuJtarJ6X_gcIat3OpOiqEXsQUtdkTPwqfgFKdqdLC3KTKVgXBEO414lti0gEDtz3AQgFBSjs4gNcBmVrDjGbpFdwuncraTXsh43A5Z8IoR9x0a7e2-iJ_WbhS_vZEOHCQa88M55UWP1D9x6e0AoNuw
Decoding the output JWT to test
Test your generated JWT by decoding it using https://jwt.io
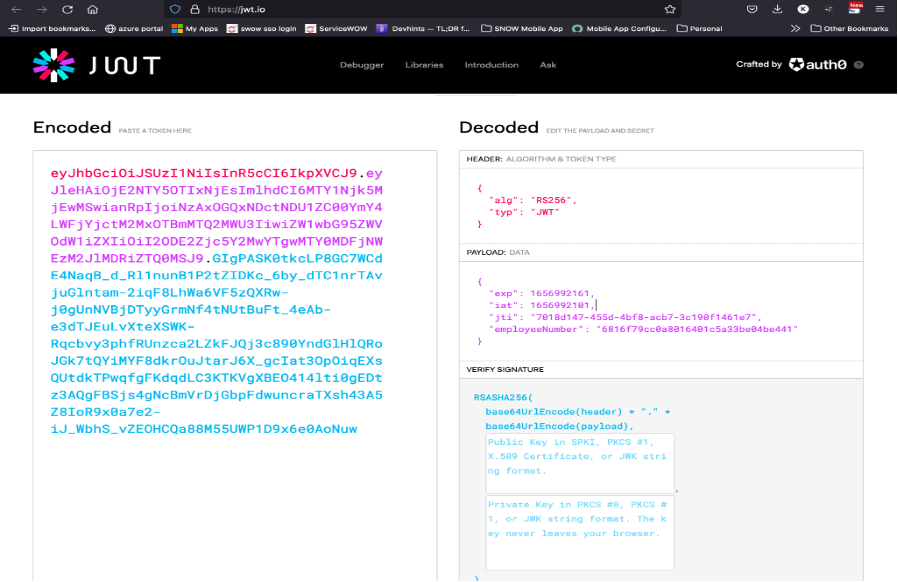